What Does “Broken JavaScript Error” Mean?
A “Broken JavaScript Error” generally refers to a situation where the JavaScript code in a web application or website is not functioning correctly. This can result in various issues, such as parts of the website not loading, interactive elements not working, or the entire page failing to render properly. Here are some common causes and explanations for a broken JavaScript error:
- Syntax Errors: These occur when there is a mistake in the code’s syntax. For example, missing a semicolon, unclosed brackets, or incorrect use of quotes can cause the script to fail.
- Reference Errors: These happen when the code tries to access a variable, function, or object that hasn’t been defined or is out of scope. For example, trying to use a variable that hasn’t been declared.
- Type Errors: These occur when a value is not of the expected type. For instance, trying to call a method on an undefined variable or perform operations on incompatible types.
- Network Errors: If the JavaScript code relies on external resources (like APIs, libraries, or assets) and these resources are unavailable or fail to load, it can break the script.
- Runtime Errors: These problems occur while the code is executing. They can be caused by logical issues, infinite loops, or unexpected inputs that the code doesn’t handle properly.
- Library/Dependency Issues: Problems can arise if the JavaScript code relies on external libraries or frameworks that are not loaded correctly, outdated, or incompatible.
- Browser Compatibility: Different browsers may interpret JavaScript code differently. Code in one browser might not work in another, leading to broken functionality.
- DOM Manipulation Errors: When JavaScript tries to manipulate the Document Object Model (DOM) but encounters elements that don’t exist or are not yet loaded, it can cause mistakes.
What Triggers JavaScript Errors?
JavaScript errors can be triggered by a variety of situations during the execution of a script. Here are some common triggers of JavaScript problems:
1. Syntax Errors
These occur when the code violates the grammatical rules of the JavaScript language.
- Missing or misplaced punctuation: e.g., if (x = 3 {} instead of if (x == 3) {}.
- Incorrect use of reserved words: e.g., using for as a variable name.
2. Runtime Errors
These occur while the script is running, often due to operations that are not possible to perform.
- ReferenceError: Trying to use a variable that hasn’t been declared, e.g., console.log(nonExistentVariable);.
- TypeError: Performing an operation on an incompatible type, e.g., null.length or undefined.someFunction().
- RangeError: Passing a number outside the allowable range, e.g., new Array(-1).
- URIError: Improper use of URI handling functions, e.g., decodeURIComponent(‘%’).
3. Logical Errors
These occur when the code doesn’t do what the developer intended, often leading to unexpected behavior.
- Off-by-one errors: Iterating one too many or one too few times.
- Incorrect conditionals: Using == instead of === or vice versa.
- Misplaced parentheses: Changing the order of operations in a way that produces incorrect results.
4. Event Handling Errors
These occur when handling user interactions or other events.
Incorrectly referencing an event target, e.g., event.target.value for a non-existent property. Attaching an event listener to a non-existent element.
5. Network Errors
These occur during interactions with external resources, such as making HTTP requests.
Network issues or invalid responses when using fetch or XMLHttpRequest. Attempting to access resources from a different domain without proper CORS headers.
6. API Errors
These occur when using JavaScript APIs incorrectly.
- DOM manipulation errors: Attempting to access or modify elements that don’t exist, e.g., document.getElementById(‘nonExistent’).innerHTML = ‘Hello’;.
- Web API usage: Using Web APIs incorrectly, such as calling canvas.getContext(‘2d’) on a non-canvas element.
7. External Library Errors
These occur when there are issues with third-party libraries or frameworks. Different libraries conflicting with each other. Using a library’s API incorrectly, e.g., passing the wrong arguments.
8. Security Errors
These occur when there are security-related issues. Malicious code injected into the site, potentially causing script errors. Violating CSP rules can block script execution.
9. Asynchronous Errors
These occur with asynchronous operations like callbacks, promises, and async/await. Failing to handle promise rejections properly. Nested callbacks leading to difficult-to-trace issues.
How to Check the Issue
Run a report to catch JavaScript files marked with 4xx or 5xx HTTP status codes. These are the files that have errors keeping the search engines from doing a proper render of the image or JavaScript files. You can also check your website yourself to see if any files or web pages show up with a 4xx or 5xx code.
On this dashboard of the Sitechecker SEO tool, you’ll find a streamlined interface that highlights various website health metrics. One crucial category here is labeled “Internal,” where specific URL-related issues are meticulously categorized for your convenience. Within this section, you’ll notice an entry marked “Page has broken JavaScript files.”
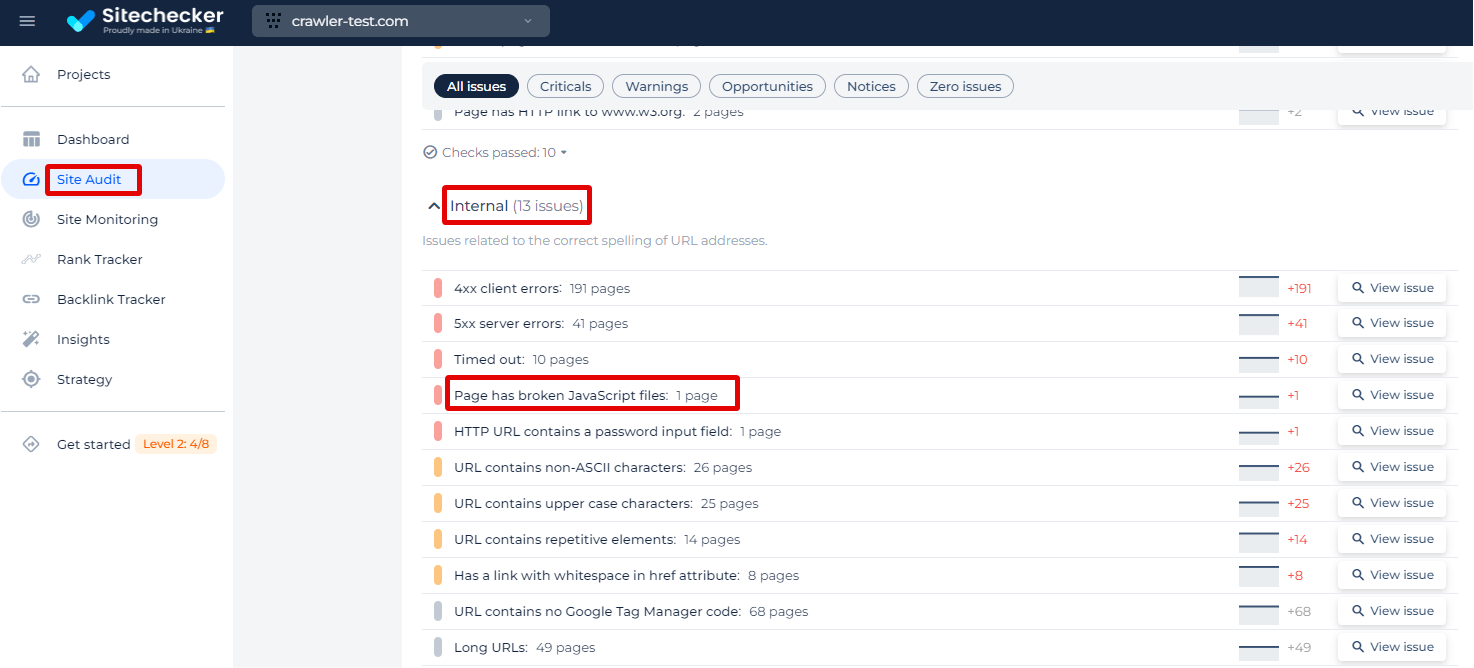
By simply clicking on the “View issue” link next to the “Page has broken JavaScript files” entry, you will be provided with a detailed list of pages that have this problem. When you explore the issue, the tool not only identifies the number of affected URLs but also provides a detailed breakdown.
For each URL listed, you’ll see essential information like the ‘Page Weight’ which gives an indication of the page’s size and potential load time, the ‘Status Code’ which indicates the HTTP response and can help identify server-side problems, and the date when the ‘Issue Found’, helping you track when the problem was detected.
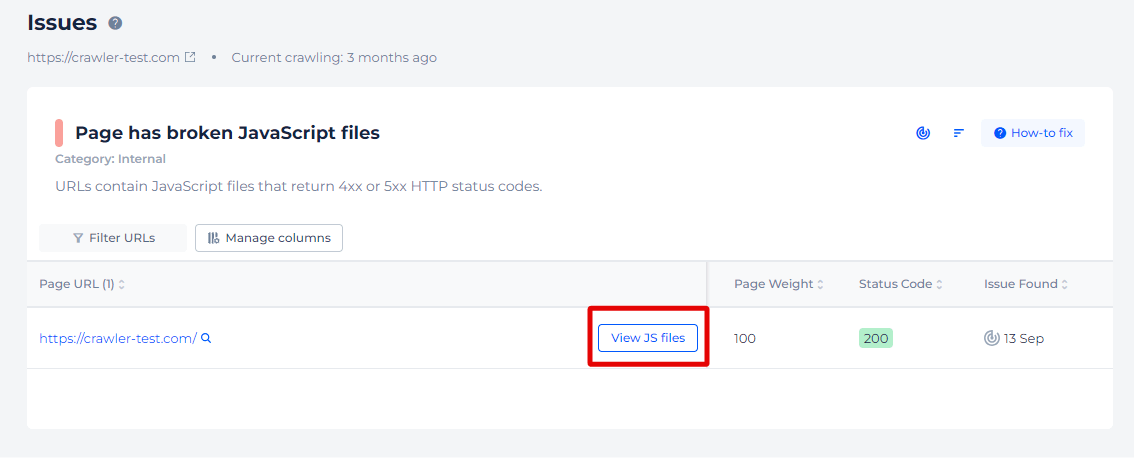
By clicking on “View JS files,” you can delve into a specific list of the JavaScript files causing errors.
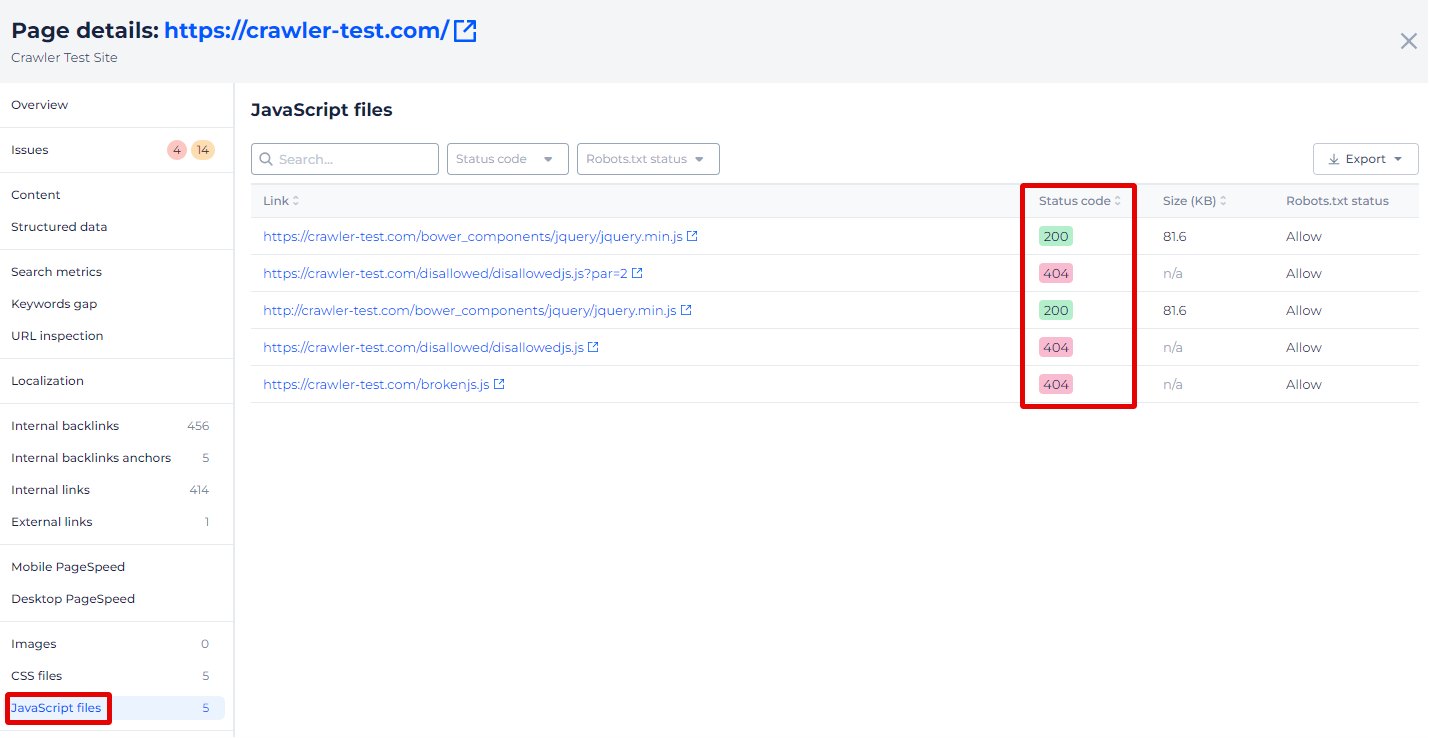
Find and Fix Broken JavaScript Instantly!
Navigate JavaScript troubles with our comprehensive checker.
How to Fix JavaScript Errors
Fixing JavaScript issues involves identifying the type of error, understanding its cause, and implementing a solution. Here’s a systematic approach to fixing common JavaScript errors:
1. Syntax Errors
Syntax errors are typically flagged by the browser or development environment with specific problem messages.
Check punctuation: Ensure all brackets, parentheses, and quotes are properly closed.
// Incorrect
if (x == 3 {
// Correct
if (x == 3) {
Correct reserved words usage: Avoid using reserved words as identifiers
// Incorrect
var for = 5;
// Correct
var count = 5;
2. Reference Errors
These issues occur when the code references a variable that hasn’t been declared.
Declare variables properly: Ensure all variables are declared before use.
// Incorrect
console.log(nonExistentVariable);
// Correct
var nonExistentVariable = 42;
console.log(nonExistentVariable);
3. Type Errors
Type errors occur when an operation is performed on a value of the wrong type.
Check types before operations: Ensure values are of the expected type.
// Incorrect
var num = null;
console.log(num.length);
// Correct
if (num !== null && num !== undefined) {
console.log(num.length);
}
4. Range Errors
Range issues occur when a value is not within the allowed range.
Validate values: Check that values fall within the acceptable range.
// Incorrect
var arr = new Array(-1);
// Correct
var size = 5;
if (size > 0) {
var arr = new Array(size);
}
5. Event Handling Errors
Problems in event handling often occur due to incorrect target references or missing elements.
Check element existence: Ensure the target elements exist before attaching event listeners.
// Incorrect
document.getElementById('nonExistent').addEventListener('click', function() {});
// Correct
var elem = document.getElementById('existent');
if (elem) {
elem.addEventListener('click', function() {});
}
6. Network Errors
Network issues occur during interactions with external resources, such as making HTTP requests.
Handle network failures gracefully: Use try-catch blocks and proper error handling.
// Incorrect
fetch('/api/data').then(response => response.json()).then(data => {
console.log(data);
});
// Correct
fetch('/api/data')
.then(response => {
if (!response.ok) throw new Error('Network response was not ok');
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
7. API Errors
API errors occur when using JavaScript APIs incorrectly.
Follow API documentation: Ensure you’re using APIs as intended.
// Incorrect
var canvas = document.getElementById('myCanvas');
var ctx = canvas.getContext('3d');
// Correct
var ctx = canvas.getContext('2d');
8. External Library Errors
These errors often result from incorrect use or conflicts between libraries.
Read library documentation: Use the library according to its documentation.
// Incorrect
$(document).ready(function() {
// Code using an old version of a library
});
// Correct
$(function() {
// Updated code according to the latest library version
});
9. Security Errors
Security errors often occur due to improper handling of user input or violating security policies.
// Incorrect
var userInput = '<script>alert("XSS")</script>';
document.getElementById('output').innerHTML = userInput;
// Correct
var safeInput = document.createTextNode(userInput);
document.getElementById('output').appendChild(safeInput);
10. Asynchronous Errors
Asynchronous errors occur with promises, callbacks, and async/await operations.
Handle promises properly: Use .catch to handle promise rejections.
// Incorrect
someAsyncFunction().then(result => {
console.log(result);
});
// Correct
someAsyncFunction()
.then(result => console.log(result))
.catch(error => console.error('Error:', error));